Describe the concepts, benefits, or operation of data serialization
-YAML
-JSON
-Jinja2
-XML
Jinja2
Jinja2 Templates (variables are generally supplied using a python data structure, often stored as YAML)
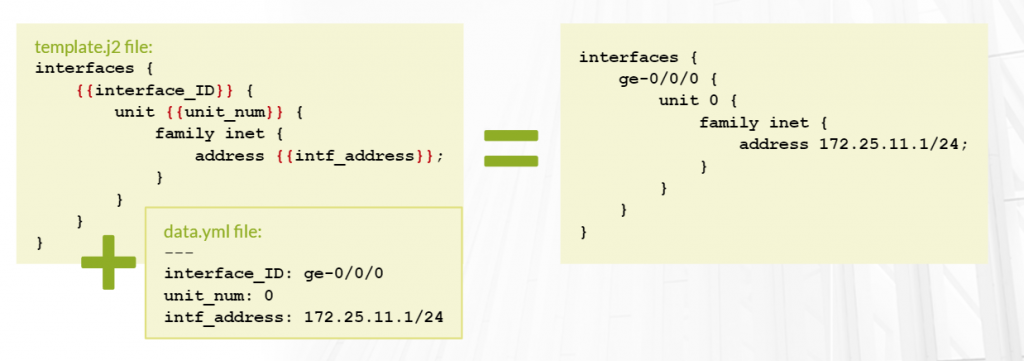
Jinja2 is a user friendly templating language for Python, modelled after Django templates.
#note this has to be run from the same file path the code is stored in.
from jinja2 import Environment, FileSystemLoader
from yaml import full_load
env = Environment(loader=FileSystemLoader('.'))
my_template = env.get_template("template.j2")
with open("data.yml") as f:
data = full_load(f)
result = my_template.render(data)
print(result)
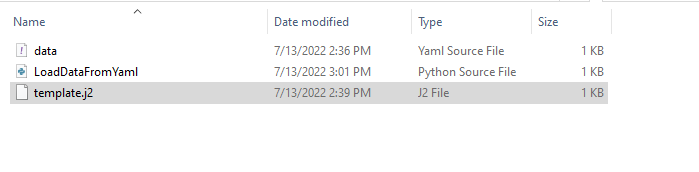
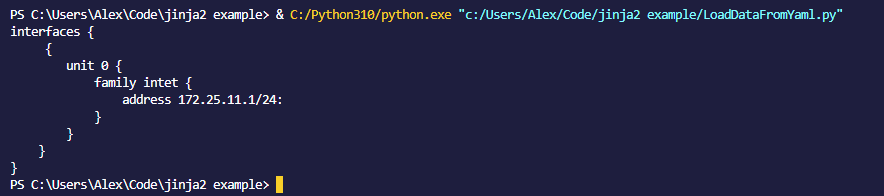
{# By default this is a comment not to be included in the output #}
{% for item in list %}
...
{% endfor %}
{{ variable_to_be_passed_in }}
Main Features of Jinja2:
Sandboxed Execution
Automatica HTML esaping system for XSS prevention
Template Inheritance
Compiles down to the optimal Python code just in time
Optional ahead-of-time template compilation
Easy to debug. Line numbers of exceptions directly point to the correct line in the template
Configurable syntax