PyEZ (Advanced)
PyEZ is a microframework for the management and automation of Junos devices.
Connecting to device using PyEZ:
SSH
dev = Device(host=hostname, user=username, passwd=password)
Telnet:
dev = Device(host=hostname, user=username, passwd=password, mode='telnet', port ='6001')
If connecting to a Terminal server the host address will be the address of the terminal server.
Direct Serial Console Connection:
dev = Device(host=hostname, user=username, passwd=password, mode='serial', port='/dev/ttyUSB0')
SSH connection through a console server:
dev = Device(host=hostname, user=username, passwd=password, cs_user='xxx', cs_passwd='xxx')
Outbound SSH is also supported (Need to work out how)
Connecting to a device through PyEZ without using SSH Key Agent:
The passwd parameter can hold the passphrase when using SSH keys:
dev = Device(host=hostname, user=username, passwd=passphrase)
If the ssh key does not have a passphrase you can omit the passwd parameter. If you want to use an ssh key that is not in the default location / has a different name you can do so too using the ssh_private_key parameter
dev = Device(host=hostname, user=username, passwd=passphrase, ssh_private_key_file='/home/user/.ssh/myfile')
If you want to find out the rpc for a command you can do that using an rpc too!
print(dev.display_xml_rpc('show interfaces terse', format='text'))
You can use specific parameters to simulate the output of more specific commands, for example:
dev.rpc.get_interface_information(interface_name='lo0', terse=True)
If a Junos command does not have an RPC equivalent, you can use the Device.cli() method. (can be used to execute any command but is recommended that it’s only used for commands without an RPC equivalent)
Exaqmple RPC into a PyEZ call:
<get-route-information>
<table> inet.0 </table>
<get-route-information>
PyEZ call:
dev.rpc.get_route_information(table="inet.0")
jxmlease is a Juniper developed library that turns XML straight into pytion objects, so you don’t have to mess around with xpath at all.
check pages 37-39 for a script that updates software that you can steal 🙂
Views and templates docs
turns the xml straight into a data object too.
JANPy
Juniper Snapshot Administrator for Python. It uses NETCONF to communicate with the Juniper devices on your network. It has two main purposes:
-To regularly check the health of devices against a known good state
-Perform a snapshot before and after maintenance windows, compare the two outputs and make sure that only the things you’ve intended to change on the device has been changed.
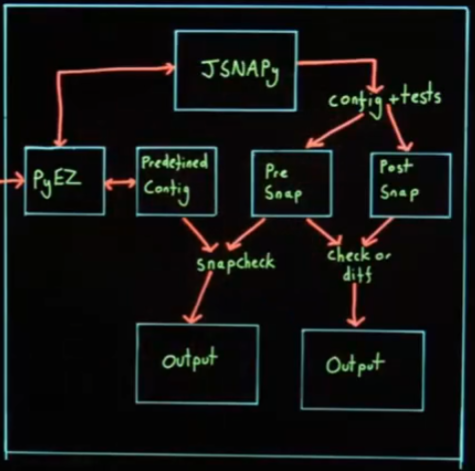
JSNAPy configuration files are in stored in YAML format, allowing you to specify which devices you want to inspect and which tests you want to run.
Jinja2
To install jinja2:
pip install jinja2
Load template from a string using the Template class from the jinja2 package
from jinja2 import Template
template_string = "Hello {{ user }}"
my_template = Template(template_string)
result = my_template.render(
{"user": "lab"}
)
print(result)